A Filter is a function that can be hooked to an event in WordPress (called hooks). During the execution when the event is triggered the filter is applied to the data output generated by the event hook.
It is important to remember that filters perform their actions on the data they receive and then return that data before it is displayed in the browser.
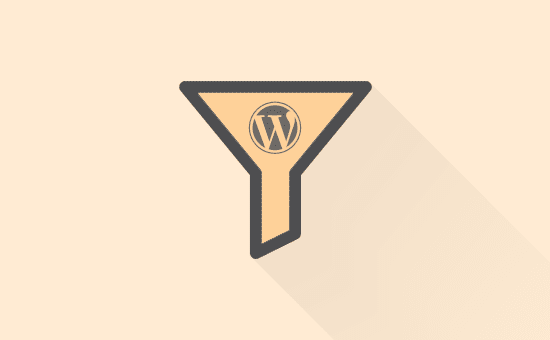
Filters always have to have data coming in and data going out to ensure the data is output in the browser (your content may be passed through other filters before getting output in the browser). By comparison, actions, which are similar to filters, do not require that anything to be returned, although data can be returned through actions as well.
Example: Let’s say we want to display an image icon when a post belongs to a particular category is displayed. In this scenario, we create a function that checks if a post is in that particular category. If it is, then display the image.
Next, we hook that function into the_content
event. Now whenever the event the_content takes place, our functional is automatically triggered to filter the output of the_content event.
// First we hook our own function with the_content event
add_filter( 'the_content', 'wpb_content_filter' );
// Now we define what our function would do.
// In this example it displays an image if a post is in news category.
function wpb_content_filter( $content ) {
if ( in_category('news') )
$content = sprintf('<img class="news-icon" src="%s/images/news_icon.png" alt="News icon" title="" />%s', get_bloginfo( 'stylesheet_directory' ), $content);
// Returns the content.
return $content;
}
Basically, filters are functions that can be used in WordPress to pass data through. They allows developers to modify the default behavior of a specific function.
Functions used to filter data are called hooks. Filters and actions together allow developers great flexibility to modify default WordPress events, filters, and actions. Developers can also create their own custom filters and actions so that other developers can extend their plugins or themes.
Filters are different than Actions. WordPress actions are executed at events like when a theme or plugin is activated, or when a post is published. Filters are used to filter output when it is sent to either database or to a user’s browser.
Another example of using a WordPress filter:
function wpb_custom_excerpt( $output ) {
if ( has_excerpt() && ! is_attachment() ) {
$output .= wpb_continue_reading_link();
}
return $output;
}
add_filter( 'get_the_excerpt', 'wpb_custom_excerpt' );
The sample code above adds a function wpb_custom_excerpt
to the filter get_the_excerpt
.
WordPress plugin API has an extensive list of filter hooks available in WordPress.
This post was originally published in the wpbeginner glossary.